How Builders Can Make improvements to Their Debugging Techniques By Gustavo Woltmann
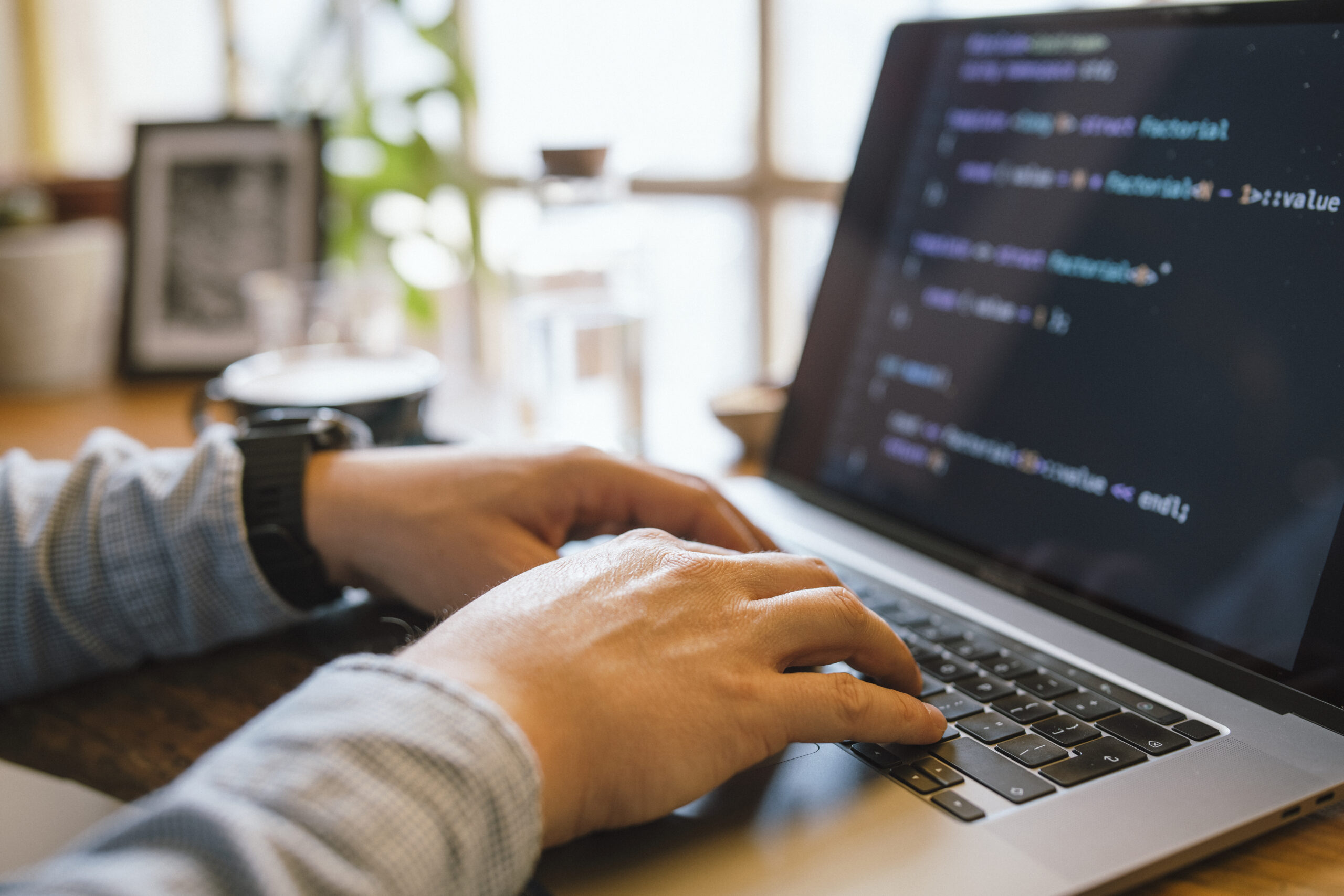
Debugging is One of the more important — nevertheless normally overlooked — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go Completely wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and drastically boost your productivity. Listed here are a number of strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is a single A part of enhancement, figuring out ways to communicate with it efficiently during execution is Similarly crucial. Modern-day growth environments come Geared up with strong debugging capabilities — but quite a few developers only scratch the surface of what these instruments can do.
Take, one example is, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in many cases modify code around the fly. When made use of accurately, they let you notice exactly how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check network requests, watch genuine-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Variation Manage techniques like Git to be aware of code record, obtain the exact moment bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your improvement atmosphere in order that when troubles come up, you’re not dropped at nighttime. The higher you understand your equipment, the more time you'll be able to devote solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more important — and sometimes disregarded — measures in efficient debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders want to create a consistent natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a game of prospect, typically leading to squandered time and fragile code improvements.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
As soon as you’ve collected ample information, endeavor to recreate the issue in your neighborhood atmosphere. This might imply inputting a similar facts, simulating equivalent user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These checks not just help expose the challenge but also avert regressions in the future.
At times, The difficulty could be ecosystem-certain — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, along with a methodical strategy. But as soon as you can continuously recreate the bug, you're already halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more successfully, check prospective fixes securely, and talk a lot more Obviously using your crew or end users. It turns an summary grievance into a concrete challenge — and that’s where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are frequently the most useful clues a developer has when one thing goes Improper. As opposed to viewing them as irritating interruptions, developers should really master to take care of error messages as direct communications from the system. They normally show you what exactly occurred, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Begin by examining the concept very carefully and in comprehensive. Quite a few developers, particularly when under time tension, glance at the very first line and straight away start out producing assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic error? Will it place to a specific file and line range? What module or perform brought on it? These inquiries can guide your investigation and position you toward the dependable code.
It’s also useful to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and learning to recognize these can drastically quicken your debugging system.
Some mistakes are imprecise or generic, As well as in Individuals scenarios, it’s essential to look at the context in which the error transpired. Check linked log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These normally precede bigger troubles and supply hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues more quickly, lessen debugging time, and turn into a extra efficient and assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of proficiently, it offers authentic-time insights into how an software behaves, encouraging you have an understanding of what’s happening under the hood with no need to pause execution or stage throughout the code line by line.
An excellent logging approach commences with being aware of what to log and at what degree. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Information for common events (like successful get started-ups), Alert for likely concerns that don’t break the applying, ERROR for real problems, and Lethal if the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your program. Concentrate on vital functions, state variations, input/output values, and critical final decision points in your code.
Structure your log messages clearly and continuously. Contain context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in generation environments exactly where stepping by code isn’t feasible.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a effectively-assumed-out logging method, it is possible to lessen the time it will take to identify concerns, get further visibility into your applications, and Enhance the In general maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method just like a detective resolving a secret. This mindset assists break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the signs or symptoms of the situation: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, gather as much related data as you may without the need of leaping to conclusions. Use logs, take a look at conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Question by yourself: What may be triggering this conduct? Have any adjustments just lately been manufactured for the codebase? Has this problem occurred right before less than very similar situation? The aim is usually to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed natural environment. In case you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code queries and let the effects guide you closer to the truth.
Pay shut consideration to tiny specifics. Bugs usually disguise while in the least envisioned areas—like a lacking semicolon, an off-by-1 mistake, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes might cover the real trouble, just for it to resurface later.
And finally, keep notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and help Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in elaborate methods.
Publish Assessments
Crafting tests is one of the best strategies to help your debugging skills and All round growth effectiveness. Assessments don't just help catch bugs early but also serve as a safety net that gives you self-assurance when generating alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint accurately where and when a problem occurs.
Get started with device checks, which center on unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Device checks are Specially valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of aspects of your application function alongside one another efficiently. They’re especially useful for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and beneath what circumstances.
Producing exams also forces you to definitely Feel critically regarding your code. To test a element effectively, you need to grasp its inputs, expected outputs, and edge situations. This level of comprehension Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—assisting you catch far more bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee crack, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
For those who’re caught, a good guideline is to established a timer—debug actively for 45–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your standpoint, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each bug you face is a lot more than simply a temporary setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously click here with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs will also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends may be especially impressive. No matter if it’s by way of a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as crucial parts of your growth journey. In the end, many of the very best builders aren't those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive away a smarter, extra capable developer thanks to it.
Conclusion
Bettering your debugging competencies requires time, follow, and tolerance — but the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.